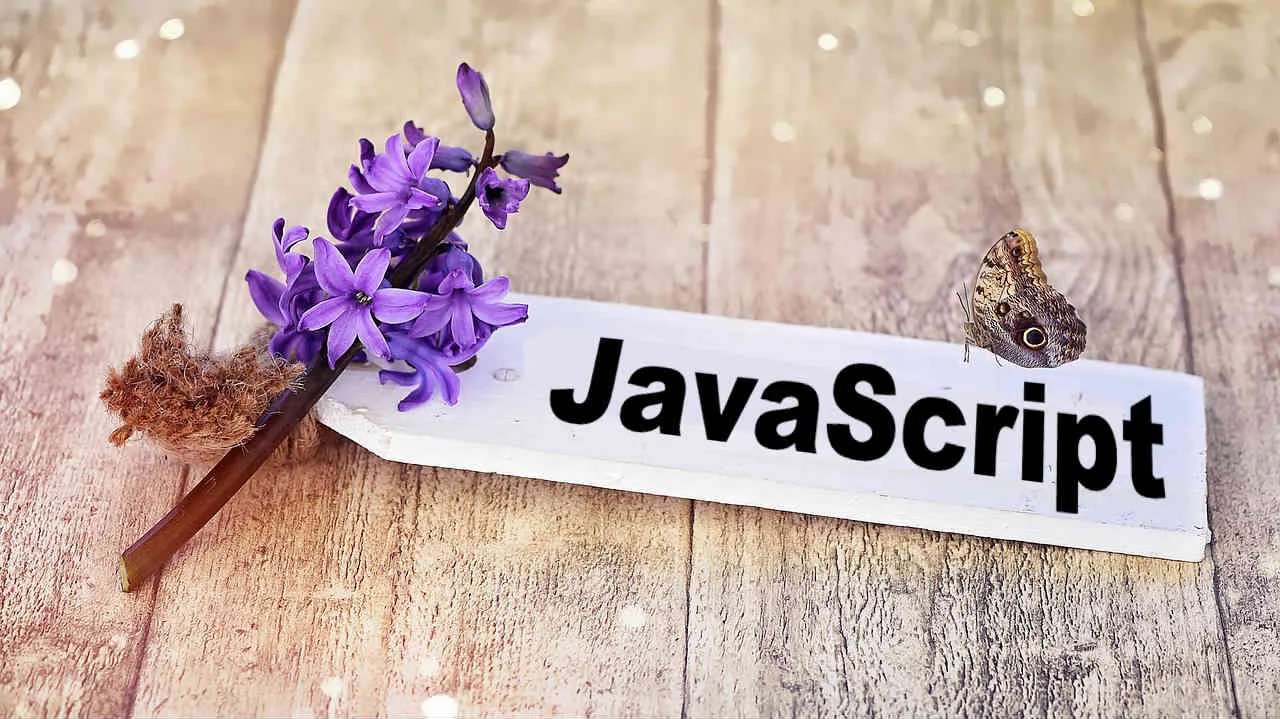
JavaScriptはブラウザ言語であるため、サーバーに設置されているファイルを読み込むのは非常に苦労します。
しかも読み込みを行う方法としては以下の方法しかありません。
1.JavaScriptファイルを読み込む
2.AJAXを利用して非同期で読み込む(ファイル直接アクセス)
3.AJAXを利用してサーバーサイド言語と連動して読み込む(他の言語連動)
サンプル
1.JavaScriptファイルを読み込む
var script = document.createElement("script");
script.type = "text/javascript";//HTML4ではこの記述は必須です。HTML5は不用になりました。
script.src = "http://hoge.com/add.js";//読み込み対象ファイルをhttpプロトコルのフルパスで記述します。
document.body.appendChild(script);
※この場合の難点は、「httpプロトコルのフルパス」でアクセスできないファイルの場合はお手上げです。
2.AJAXを利用して非同期で読み込む(ファイル直接アクセス)
var ajax = {
xmlObj:function(f){
var r=null;
try{
r=new XMLHttpRequest();
}
catch(e){
try{
r=new ActiveXObject("Msxml2.XMLHTTP");
}
catch(e){
try{
r=new ActiveXObject("Microsoft.XMLHTTP");
}
catch(e){
return null;
}
}
}
return r;
},
/**
* XMLHttpRequestオブジェクト生成
*/
set:function(option){
if(!option){return}
ajax.httpoj = ajax.createHttpRequest();
if(!ajax.httpoj){return;}
//open メソッド;
ajax.httpoj.open(option.method , option.url , option.async);
ajax.httpoj.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
//受信時に起動するイベント;
ajax.httpoj.onreadystatechange = function(){
//readyState値は4で受信完了;
if (this.readyState==4){
alert(this.responseText);
}
};
//query整形
var data = [];
if(typeof(option.query)!="undefined"){
for(var i in option.query){
data.push(i+"="+encodeURIComponent(option.query[i]));
}
}
if(typeof(option.querys)!="undefined"){
for(var i=0;i<option .querys.length;i++){
data.push(option.querys[i][0]+"="+encodeURIComponent(option.querys[i][1]));
}
}
//send メソッド
if(data.length){
ajax.httpoj.send( data.join("&") );
}
else{
ajax.httpoj.send();
}
},
createHttpRequest:function(){
//Win ie用
if(window.ActiveXObject){
try {
//MSXML2以降用;
return new ActiveXObject("Msxml2.XMLHTTP")
}
catch(e){
try {
//旧MSXML用;
return new ActiveXObject("Microsoft.XMLHTTP")
}
catch(e2){
return null
}
}
}
//Win ie以外のXMLHttpRequestオブジェクト実装ブラウザ用;
else if(window.XMLHttpRequest){
return new XMLHttpRequest()
}
else{
return null
}
}
};
ajax.set({
url:"%読み込むファイルのURL%",
methos:"post",
async:true
});
※この場合の難点も、「httpプロトコルのフルパス」でアクセスできないファイルの場合はお手上げです。
また、サーバー設定で、x-frame-optionというのをセットされていると、外部ドメインでは取得できなくなるので、要注意です。
3.AJAXを利用してサーバーサイド言語と連動して読み込む(他の言語連動)
var ajax = {
xmlObj:function(f){
var r=null;
try{
r=new XMLHttpRequest();
}
catch(e){
try{
r=new ActiveXObject("Msxml2.XMLHTTP");
}
catch(e){
try{
r=new ActiveXObject("Microsoft.XMLHTTP");
}
catch(e){
return null;
}
}
}
return r;
},
/**
* XMLHttpRequestオブジェクト生成
*/
set:function(option){
if(!option){return}
ajax.httpoj = ajax.createHttpRequest();
if(!ajax.httpoj){return;}
//open メソッド;
ajax.httpoj.open(option.method , option.url , option.async);
ajax.httpoj.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
//受信時に起動するイベント;
ajax.httpoj.onreadystatechange = function(){
//readyState値は4で受信完了;
if (this.readyState==4){
alert(this.responseText);
}
};
//query整形
var data = [];
if(typeof(option.query)!="undefined"){
for(var i in option.query){
data.push(i+"="+encodeURIComponent(option.query[i]));
}
}
if(typeof(option.querys)!="undefined"){
for(var i=0;i<option .querys.length;i++){
data.push(option.querys[i][0]+"="+encodeURIComponent(option.querys[i][1]));
}
}
//send メソッド
if(data.length){
ajax.httpoj.send( data.join("&") );
}
else{
ajax.httpoj.send();
}
},
createHttpRequest:function(){
//Win ie用
if(window.ActiveXObject){
try {
//MSXML2以降用;
return new ActiveXObject("Msxml2.XMLHTTP")
}
catch(e){
try {
//旧MSXML用;
return new ActiveXObject("Microsoft.XMLHTTP")
}
catch(e2){
return null
}
}
}
//Win ie以外のXMLHttpRequestオブジェクト実装ブラウザ用;
else if(window.XMLHttpRequest){
return new XMLHttpRequest()
}
else{
return null
}
}
};
ajax.set({
url:"import.php",
methos:"post",
async:true,
query:{"filePath":"%ファイルパス%"}
});
# import.php
<? php
file_get_contents($_REQUEST["filePath"])
※どの場合も対応できますが、サーバーサイドに読み込み用のプログラムを設置しなければいけないため、構成を熟知しておく必要があります。
※同一サーバーに設置していあるテキストファイルを読み込む事ができます。
注意点
通常のサーバーサイド限度では、readやfile_get_contentsやopenなどの関数を使って配置されているファイルを簡単に読み込むことが出来るんですが、この辺がブラウザ言語の悲しいトコロですね。
0 件のコメント:
コメントを投稿